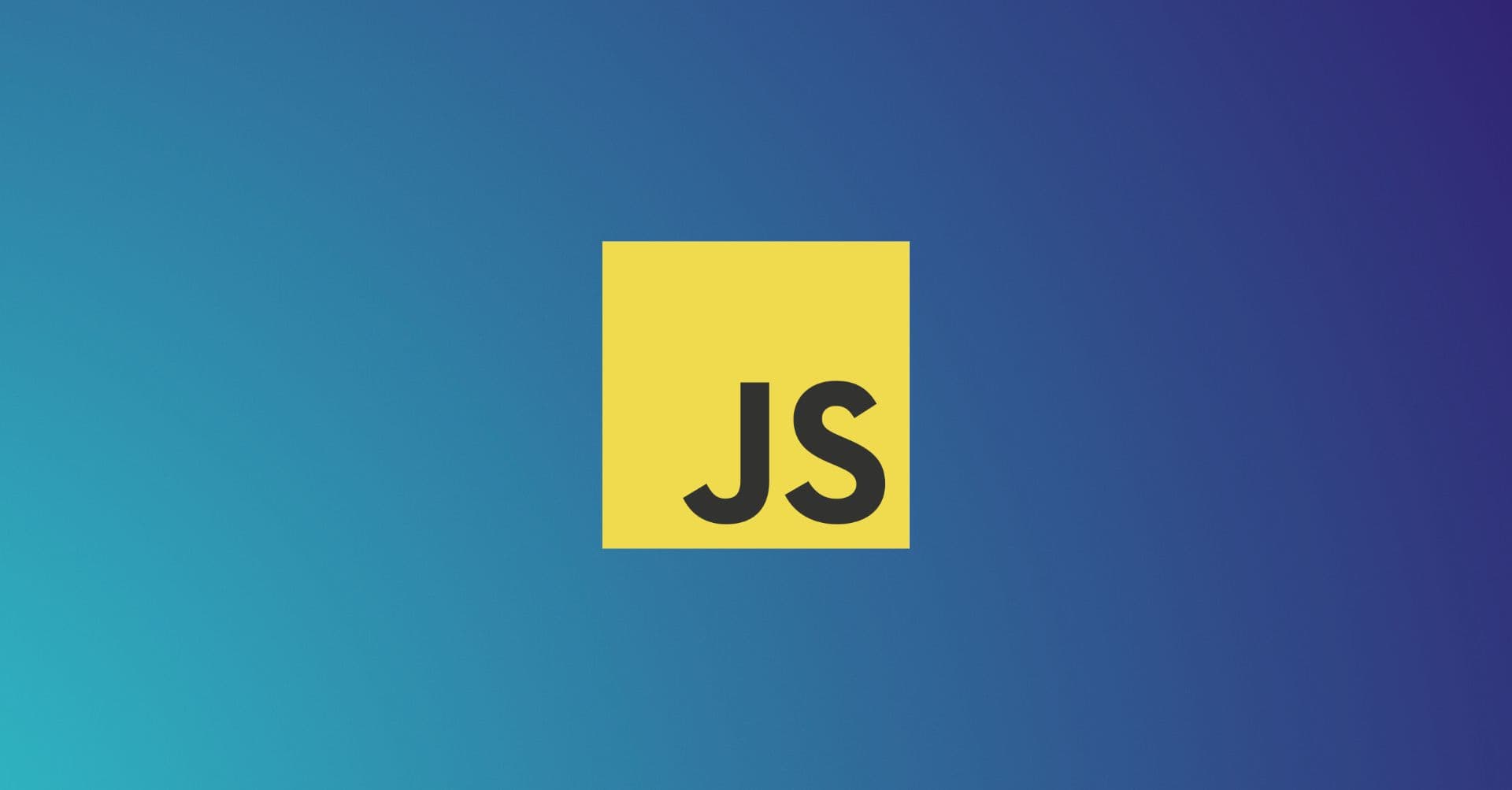
5 Must-Know Features in JavaScript ECMAScript 2024
1360 ViewsECMAScript 2024 introduces key features that enhance JavaScript. In this article, we’ll explore five updates that simplify coding and improve performance.
What is ECMAScript?
ECMAScript is a standard for scripting languages, including JavaScript, JScript, and ActionScript. It is best known as a JavaScript standard intended to ensure the interoperability of web pages across different web browsers. It is standardized by Ecma International in the document ECMA-262. ECMAScript is commonly used for client-side scripting on the World Wide Web, and it is increasingly being used for server-side applications and services using runtime environments - Node.js, deno, and bun. (Wikipedia).
Warning: Compatibility with Older Browsers
Many features in ECMAScript 2024 are new, and older browsers may not support them yet. To ensure your code works across all platforms, consider using polyfills to provide alternative solutions where needed.
Let's dive into the standout features of ECMAScript 2024, Here are the most important ones you should know about:
Object.groupBy()
Object.groupBy() is a new static method that allows you to group elements of an iterable object, such as an array, based on a callback function. This method returns an object where each key corresponds to a group determined by the callback.
Syntax:
1Object.groupBy(iterable_objects, keySelectorCallback);
Code Example:
1const cars = [
2 { name: "Toyota", type: "Sedan" },
3 { name: "Toyota", type: "SUV" },
4 { name: "Toyota", type: "Truck" },
5 { name: "BMW", type: "Sedan" },
6 { name: "BMW", type: "SUV" },
7 { name: "BMW", type: "Truck" },
8 { name: "Mercedes", type: "Sedan" },
9 { name: "Mercedes", type: "SUV" },
10 { name: "Mercedes", type: "Truck" },
11]
12
13const result = Object.groupBy(cars, ({type}) => type);
Now If we print the result object, it will look like this:
1console.log("result is:", result);
2/*
3result is: [Object: null prototype] {
4 Sedan: [
5 { name: 'Toyota', type: 'Sedan' },
6 { name: 'BMW', type: 'Sedan' },
7 { name: 'Mercedes', type: 'Sedan' }
8 ],
9 SUV: [
10 { name: 'Toyota', type: 'SUV' },
11 { name: 'BMW', type: 'SUV' },
12 { name: 'Mercedes', type: 'SUV' }
13 ],
14 Truck: [
15 { name: 'Toyota', type: 'Truck' },
16 { name: 'BMW', type: 'Truck' },
17 { name: 'Mercedes', type: 'Truck' }
18 ]
19}
20*/
As you can see, the result object uses the type values as keys. Each key corresponds to an array of objects that share the same type. To access the grouped data, you can simply refer to the desired key in the result object.
1console.log("result.Sedan is:", result.Sedan);
2
3/*
4result.Sedan is: [
5 { name: 'Toyota', type: 'Sedan' },
6 { name: 'BMW', type: 'Sedan' },
7 { name: 'Mercedes', type: 'Sedan' }
8]
9*/
The Object.groupBy() is immutable, meaning it does not modify the original object but instead creates a new one with the grouped data.
Map.groupBy()
Map.groupBy() is pretty similar to the Object.groupBy(), a new static method that allows you to group elements of an iterable object, such as an array, based on a callback function. The final returned Map contains unique values of the original iterable object's keys, where each key corresponds to a group determined by the callback.
The code example above would work similarly with Map.groupBy(), with the key difference being that the result would be a Map instead of an object. This allows for greater flexibility in the types of keys used for grouping.
The Map.groupBy() is also immutable, meaning it does not modify the original object but instead creates a new one with the grouped data.
When to use what (Map.groupBy() vs Object.groupBy())?
Use Object.groupBy() when your grouping criteria are simple and can be represented as strings or symbols.
Use Map.groupBy() when you need flexibility with key types, as Map allows keys of any type, not just strings or symbols. This method is ideal for grouping data associated with objects that might change over time.
Promise.withResolvers
Another new static method introduced in ECMAScript 2024 is Promise.withResolvers(). This method returns an object containing the promise itself, along with two functions: the resolver and rejecter. This simplifies creating and managing promises with external resolve and reject controls.
Before this, achieving similar functionality required a more manual approach. You might have written something like this:
1let resolve, reject;
2const promise = new Promise((res, rej) => {
3 resolve = res;
4 reject = rej;
5});
6
This approach works but involves extra steps to manually store the resolve and reject functions. Promise.withResolvers() streamlines this by packaging everything together in a single object:
1const {promise, resolve, reject} = Promise.withResolvers()
2
String.prototype.isWellFormed
The String.prototype.isWellFormed method is designed to check if a string is well-formed according to Unicode encoding standards. It returns a boolean value:
- true if the string does not contain any lone surrogates.
- false if the string contains one or more lone surrogates.
Code Example:
1const validString = "Hello, world! 🌍"; // Well-formed string
2console.log(validString.isWellFormed()); // true
3
4const invalidString = "Hello, world! \uD83D"; // Lone surrogate
5console.log(invalidString.isWellFormed()); // false
6
String.prototype.toWellFormed
The String.prototype.toWellFormed method is designed to ensure that a string is well-formed according to Unicode encoding standards. Specifically, it applies Unicode normalization to ensure the string contains no lone surrogates and adheres to well-formed encoding rules.
Code Example:
1const malformedString = "Hello, world! \uD83D"; // Contains a lone surrogate
2
3const wellFormedString = malformedString.toWellFormed();
4console.log(wellFormedString); // "Hello, world! �" (well-formed)
5
6const alreadyWellFormed = "Hello, world! 🌍"; // Already well-formed
7const unchangedString = alreadyWellFormed.toWellFormed();
8console.log(unchangedString); // "Hello, world! 🌍" (unchanged)
References:
- ECMAScript® 2024 Language Specification - tc39
- ECMAScript - Wikipedia
- JavaScript Object.groupBy() Method - w3schools
- JavaScript Map.groupBy() Method - w3schools
- Promise.withResolvers() - JavaScript | MDN
- String.prototype.toWellFormed() - JavaScript | MDN
- String.prototype.isWellFormed() - JavaScript | MDN
Conclusion
We’ve highlighted five key features in ECMAScript 2024, including Object.groupBy(), Map.groupBy(), and Promise.withResolvers(). These updates offer powerful new tools for JavaScript development, enhancing functionality and ease of use. As you integrate these features into your projects, keep in mind browser compatibility and immutability concerns. Embrace these innovations to stay current and improve your coding practices. Happy coding!